#
需求:從學生表中查詢出所有姓名和班級都相同的學生信息。
SQL:select distinct name,class,count(*) as recordcount from student group by name,class having count(*)>1;
將“終端服務配置”中的“授權模式”更改為“每用戶”。為此,請按照下列步驟操作:
1.單擊“開始”,“管理工具”,然后單擊“終端服務配置”。
2.單擊“服務器設置”,然后雙擊“授權模式”。
3.將“授權模式”更改為“每用戶”,然后單擊“確定”。
1.生成測試數據:
create table team(name varchar(32),person varchar(32));
insert into team values('A','Tom');
insert into team values('A','Jim');
insert into team values('A','Anny');
insert into team values('B','Ivy');
insert into team values('B','Henry');
insert into team values('C','Dar');
insert into team values('C','Rk');
insert into team values('D','Cenic');
select * from team;
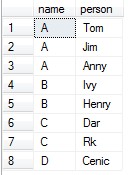
2.創建合并團隊成員姓名自定義函數:
create function fun_combName(@teamname varchar(32))
returns varchar(4000)
as
begin
declare @temp varchar(4000)
set @temp = ''
select @temp = @temp+';'+person from team where name = @teamname
set @temp = stuff(@temp,1,1,'')
return @temp
end
3.執行查詢語句:
select name,person = dbo.fun_combName(name) from team group by name order by name;
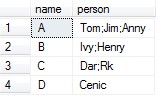
注:測試完可以刪除測試數據:
drop function fun_combName;
drop table team;
 1.從最簡單的做起,在最短的時間內完成最多的模塊,把難點放到最后,這樣可以使開發效率提高,對客戶也好反饋;
 2.不要去等待客戶,盡自己所能去做,最后即使出現問題客戶也說不出什么。
student:name,sex,age
score:name,subject,score
想要的結果:name,sex,age,sum(score)
1.
select s.name,s.sex,s.age,(select sum(c.score) from score c where c.name = s.name) from student s;
2.
select s.name,s.sex,s.age,sc.total from (select sum(c.score) as total,c.name
from score c group by c.name) sc inner join student s on s.name = sc.name;
jFreeChart是Java開發中常用的統計類組件,主要包括柱狀圖,餅狀圖等。下面我們介紹一下jFreeChart最簡單的用法。
首先需要導入jFreeChart的jar包,放在項目web\WEB-INF\lib文件夾下。然后我們以最簡潔的代碼實現一個統計功能。
1.柱狀圖
import java.awt.Color;
import java.awt.Font;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;

import org.jfree.chart.ChartFactory;
import org.jfree.chart.ChartUtilities;
import org.jfree.chart.JFreeChart;
import org.jfree.chart.axis.CategoryAxis;
import org.jfree.chart.axis.NumberAxis;
import org.jfree.chart.plot.CategoryPlot;
import org.jfree.chart.plot.PlotOrientation;
import org.jfree.chart.title.TextTitle;
import org.jfree.data.category.DefaultCategoryDataset;

 public class BarChartTest {
 public static void main(String[] args) {
DefaultCategoryDataset dataset = new DefaultCategoryDataset();
dataset.addValue(20, "企業備案數", "北京局");
dataset.addValue(18, "企業備案數", "上海局");
dataset.addValue(16, "企業備案數", "天津局");
dataset.addValue(15, "企業備案數", "重慶局");
dataset.addValue(45, "企業備案數", "山東局");
JFreeChart chart = ChartFactory.createBarChart("企業備案圖", "直屬局", "企業備案數",dataset, PlotOrientation.HORIZONTAL, true, false, false);
 /** *//***************A start*********/
//設置標題字體樣式
TextTitle textTitle = chart.getTitle();
textTitle.setFont(new Font("黑體", Font.PLAIN, 20));
//設置柱狀體顏色
CategoryPlot categoryplot = (CategoryPlot) chart.getPlot();
categoryplot.getRenderer().setSeriesPaint(0, new Color(228,109,10));
NumberAxis numberaxis = (NumberAxis) categoryplot.getRangeAxis();
CategoryAxis domainAxis = categoryplot.getDomainAxis();
//設置X軸坐標上的字體樣式
domainAxis.setTickLabelFont(new Font("sans-serif", Font.PLAIN, 11));
//設置X軸的標題字體樣式
domainAxis.setLabelFont(new Font("宋體", Font.PLAIN, 12));
//設置Y軸坐標上的字體樣式
numberaxis.setTickLabelFont(new Font("sans-serif", Font.PLAIN, 12));
//設置Y軸的標題字體樣式
numberaxis.setLabelFont(new Font("黑體", Font.PLAIN, 12));
//設置圖片最底部字體樣式
 if (chart.getLegend() != null) {
chart.getLegend().setItemFont(new Font("宋體", Font.PLAIN, 12));
}
 /** *//***************A end*********/
 try {
ChartUtilities.writeChartAsPNG(new FileOutputStream("D:\\barChart.jpg"), chart, 400, 200);
 } catch (FileNotFoundException e) {
e.printStackTrace();
 } catch (IOException e) {
e.printStackTrace();
}
}
}

生成的文件顯示效果如下:

2.餅狀圖
import java.awt.Color;
import java.awt.Font;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.text.DecimalFormat;
import java.text.NumberFormat;

import org.jfree.chart.ChartFactory;
import org.jfree.chart.ChartUtilities;
import org.jfree.chart.JFreeChart;
import org.jfree.chart.labels.StandardPieSectionLabelGenerator;
import org.jfree.chart.plot.PiePlot;
import org.jfree.data.general.DefaultPieDataset;

 public class PieChartTest {
 public static void main(String[] args) {
DefaultPieDataset pieDataset = new DefaultPieDataset();
pieDataset.setValue("北京局",20);
pieDataset.setValue("上海局",18);
pieDataset.setValue("天津局",16);
pieDataset.setValue("重慶局",15);
pieDataset.setValue("山東局",45);
JFreeChart chart = ChartFactory.createPieChart3D("企業備案圖",pieDataset, true, false, false);
 /** *//***************A start*********/
//設置標題字體樣式
chart.getTitle().setFont(new Font("黑體",Font.BOLD,20));
//設置餅狀圖里描述字體樣式
PiePlot piePlot= (PiePlot) chart.getPlot();
piePlot.setLabelFont(new Font("黑體",Font.BOLD,10));
//設置顯示百分比樣式
piePlot.setLabelGenerator(new StandardPieSectionLabelGenerator(
("{0}({2})"), NumberFormat.getNumberInstance(),
new DecimalFormat("0.00%")));
//設置統計圖背景
piePlot.setBackgroundPaint(Color.white);
//設置圖片最底部字體樣式
chart.getLegend().setItemFont(new Font("黑體",Font.BOLD,10));
 /** *//***************A end*********/
 try {
ChartUtilities.writeChartAsPNG(new FileOutputStream("D:\\pieChart.jpg"), chart, 400, 300);
 } catch (FileNotFoundException e) {
e.printStackTrace();
 } catch (IOException e) {
e.printStackTrace();
}
}

}

生成的文件顯示效果如下:
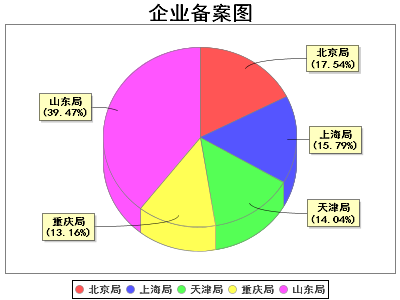
其中以上兩段代碼中的“A”代碼塊中的內容是為了解決圖片生成過程中遇到的亂碼問題,在實際開發中可以寫到一個公共類中,此時應注意服務器的操作系統上是否缺少上述代碼中所用到的字體。關于jFreeChart詳細參數的使用請參考官方文檔。
今天去總局部署項目了,經歷實在坎坷。上午一到那,值班人員是臨時接替的,不熟悉機房的環境,找顯示器,找鍵盤,插接口,慢慢開始適應環境。一切準備就緒,開始裝系統,系統順利的裝完了,可配IP地址出問題了,找不到網絡鄰居的本地連接,打了N個電話,最后確認,網卡沒有驅動起來,這就開始了尋找合適網卡的艱難歷程,從11:30到16:30五個小時,終于在機房維護人員與HP客服的協助下成功裝上驅動,一切正常了,離工作人員下班只有半小時了,趕緊開始裝軟件部署環境,oracle9i安裝出錯了,沒時間查找錯誤原因,還好帶了一套10g,換裝oracle10g,服務器很快裝好,但客戶端配置出現了問題,一樣來不及找原因,換sqldeveloper,導數據,啟動服務,部署項目,天哪,終于搞定了!總結一下去機房部署要注意的事項:
1.帶上一個以上筆記本電腦;
2.帶上USB鼠標,最好還能有USB鍵盤,小型顯示器;
3.帶上一根以上網線;
4.帶上圓口轉USB接口的轉接頭;
5.提前想好一切有可能發生的事情。
簡單總計一下今天的經歷,呵呵。
Java web程序中備份oracle數據庫
1.生成備份文件:
public ActionForward createDmp(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response)
 throws Exception {
Calendar c = Calendar.getInstance();
SimpleDateFormat sdf = new SimpleDateFormat("yyyyMMddHHmmss");
String fileName = "data_oracle" + sdf.format(c.getTime());
String dmpFolder = request.getSession().getServletContext()
.getRealPath("")
+ "\\datas\\dmp\\";
String logFolder = request.getSession().getServletContext()
.getRealPath("")
+ "\\datas\\log\\";

String cmd = "exp username/password@orcl file='" + dmpFolder + fileName
+ ".dmp' log='" + logFolder + fileName + ".log' owner=username";
Runtime.getRuntime().exec(cmd);
Thread.sleep(100 * 1000);
request.setAttribute("msg", "備份成功,文件生成會有延遲,請稍等");
return mapping.findForward("dmpCreated");
}
2.查詢備份文件:
public ActionForward findDmp(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response)
 throws Exception {
String dmpFolder = request.getSession().getServletContext()
.getRealPath("")
+ "\\datas\\dmp\\";
File folder = new File(dmpFolder);
File[] files = folder.listFiles();
List<String[]> fileList = new ArrayList<String[]>();
 for (int i = 0; i < files.length; i++) {
 if (files[i].getName().indexOf(".dmp") > 0) {
String[] one = new String[2];
one[0] = files[i].getName();
one[1] = files[i].length() / 1000 + "K";
fileList.add(one);
}
}
request.setAttribute("fileList", fileList);
return mapping.findForward("dmpList");
}
3.刪除備份文件:
public ActionForward deleteDmp(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response)
 throws Exception {
String dmpFolder = request.getSession().getServletContext()
.getRealPath("")
+ "\\datas\\dmp\\";
File folder = new File(dmpFolder);
File[] files = folder.listFiles();
String fileName = request.getParameter("dmpName");
 for (int i = 0; i < files.length; i++) {
 if (files[i].getName().equals(fileName)) {
files[i].delete();
request.setAttribute("msg", "刪除成功");
break;
}
}
return mapping.findForward("dmpList");
}
|