簡單分欄式變換加密技術(shù):
? (1)將明文消息一行一行寫入預定長度的矩形中
? (2)一列一列讀消息,但不一定按1、2、3列的順序,也可以按隨機順序,如2、3、1
? (3)得到的消息就是密文消息
? 注:密鑰為字符的話,就根據(jù)ASII碼的大小進行組合。
? 實現(xiàn)的算法:按照排序好的數(shù)組bufClone的字符遍歷尋找對應buf的字符的位置,然
? 后取出該位置整數(shù)倍的位置的字符
? SimpleColumar.java?1?package?SimpleColumnarTransposition;
?2?import?javax.swing.JFrame;
?3?public?class?SimpleColumar?{
?4?????public?static?void?main(String[]?args)?{
?5?????????//實例化一個窗體
?6?????????SimpleColumnarFrame?frame?=?new?SimpleColumnarFrame();
?7?????????frame.setVisible(true);
?8?????????frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
?9?????}????
10?}
11?
12?
?
SimpleColumnarFrame.java??1?package?SimpleColumnarTransposition;
??2?
??3?import?java.awt.event.ActionEvent;
??4?import?java.awt.event.ActionListener;
??5?import?java.util.Arrays;
??6?
??7?import?javax.swing.*;
??8?
??9?@SuppressWarnings({?"static-access",?"static-access",?"static-access",?"static-access"?})
?10?public?class?SimpleColumnarFrame?extends?JFrame?{
?11?????JButton?btnKey?=?new?JButton();
?12?????JButton?btnReset?=?new?JButton();
?13?????JLabel?lbTest?=?new?JLabel();
?14?????JLabel?lbKey?=?new?JLabel();
?15?????JPasswordField?jpf?=?new?JPasswordField();
?16?????JTextField?tfDarkPass?=?new?JTextField();
?17?????JTextField?tfKey?=?new?JTextField();
?18?????char[]?arr;
?19?????byte[]?buf,bufClone;
?20?????
?21?????public?SimpleColumnarFrame()?{
?22?????????this.setSize(300,200);????????//設置窗體大小
?23?????????this.setTitle("簡單變換柵欄加密法");
?24?????????this.setResizable(false);????//設置窗體大小不可變
?25?????????Init();????????//填充窗體內(nèi)容
?26?????}
?27?????
?28?????
?29?????private?void?makeBrightText()?{
?30?????????tfDarkPass.setEditable(true);
?31?????????arr?=?jpf.getPassword();?????//獲取明文
?32?????????buf?=?tfKey.getText().getBytes();?????//獲取密鑰,用ASII碼進行比較
?33?????????bufClone?=?buf.clone();?????//克隆數(shù)組
?34?????????Arrays.sort(bufClone);?????????//?根據(jù)ASII碼排序
?35?????????reform();????????//重組字符
?36?????????tfDarkPass.setEditable(false);
?37?????}
?38?????
?39?????private??void?reform()?{
?40?????????/*?按照排序好的數(shù)組bufClone的字符遍歷尋找對應buf的字符的位置,然
?41????????????后取出該位置整數(shù)倍的位置的字符*/
?42?????????char[]?a?=?new?char[arr.length];
?43?????????int?index?=?0;
?44?????????for(int?i=0;i<bufClone.length;i++)
?45?????????????for(int?j=0;j<buf.length;j++)?
?46?????????????????if(buf[j]==bufClone[i])?
?47?????????????????????for(int?k=0;k<arr.length;k++)?
?48?????????????????????????if((k+1)%buf.length==(j+1)%buf.length)
?49?????????????????????????????a[index++]?=?arr[k];????????
?50?????????String?str?=?new?String(a);
?51?????????tfDarkPass.setText(str);
?52?????}
?53?????
?54?????private?void?reset()?{
?55?????????tfDarkPass.setEditable(true);
?56?????????jpf.setText("");
?57?????????tfKey.setText("");
?58?????????tfDarkPass.setText("");
?59?????????tfDarkPass.setEditable(false);
?60?????}
?61?
?62?????private?void?Init()?{
?63?????????//????設置窗體內(nèi)容????????
?64?????????this.setLayout(null);
?65?????????lbTest.setText("輸入明文:");?
?66?????????lbTest.setBounds(30,?20,?80,?30);
?67?????????lbKey.setText("密鑰:?");
?68?????????lbKey.setBounds(30,?70,?80,?30);
?69?????????btnKey.setText("生成密文:");
?70?????????btnKey.setBounds(75,?110,?100,?30);????
?71?????????btnReset.setText("重置");
?72?????????btnReset.setBounds(5,?110,?60,?30);????
?73?????????jpf.setBounds(160,?20,?80,?30);
?74?????????jpf.setEchoChar('*');????????
?75?????????tfDarkPass.setBounds(190,?110,?80,?30);
?76?????????tfDarkPass.setEditable(false);
?77?????????tfKey.setBounds(160,?70,?80,?30);
?78?????????
?79?????????this.add(lbTest);
?80?????????this.add(jpf);
?81?????????this.add(lbKey);
?82?????????this.add(btnKey);
?83?????????this.add(btnReset);
?84?????????this.add(tfDarkPass);
?85?????????this.add(tfKey);
?86?????????
?87?????????btnKey.addActionListener(new?ActionListener(){
?88?????????????//觸發(fā)事件,生成明文
?89?????????????public?void?actionPerformed(ActionEvent?e)?{
?90?????????????????makeBrightText();????????????
?91?????????????}
?92?
?93?????????});
?94?????????
?95?????????btnReset.addActionListener(new?ActionListener(){
?96?????????????//觸發(fā)事件,生成明文
?97?????????????public?void?actionPerformed(ActionEvent?e)?{
?98?????????????????reset();????//????重置????
?99?????????????}
100?
101?????????});
102?????}
103?????
104?}
105?
?現(xiàn)實圖解:
?
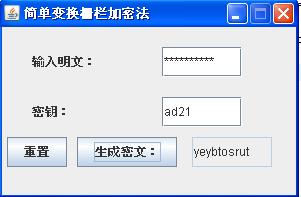
?輸入明文及密鑰,點擊生成密文按鈕,則產(chǎn)生相應密文,重置,則重新填寫相關(guān)信息。
?