線程回調(diào)方式我們已經(jīng)在"
使用回調(diào)和線程處理一個(gè)耗時(shí)響應(yīng)過(guò)程"文中進(jìn)行了講述,但是有些情況下用戶希望在指定時(shí)間內(nèi)返回一個(gè)結(jié)果,免得無(wú)休止的等待下去.這時(shí)我們需要使用"限時(shí)線程回調(diào)方式",它在原有線程回調(diào)的基礎(chǔ)上加上了一個(gè)Timer以計(jì)算消耗的時(shí)間,如果時(shí)間期限到了任務(wù)還沒(méi)有執(zhí)行完的話即中斷線程,示例代碼如下:
package com.sitinspring;

import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;

import javax.swing.Timer;


/** *//**
* 定時(shí)回調(diào)線程類(lèi)
*
* @author sitinspring(junglesong@gmail.com)
*
* @date 2007-11-6
*/

public class TimedCallBackThread implements Runnable
{
// 一秒的毫秒數(shù)常量
private final static int ONE_SECOND = 1000;

// 限制時(shí)間,以秒為單位
private final int waitTime;

// 已經(jīng)流逝的時(shí)間
private int passedTime;

private Timer timer;

private Thread thread;

private MvcTcModel model;

private MvcTcView view;


public TimedCallBackThread(MvcTcModel model, MvcTcView view, int waitTime)
{
this.model = model;
this.view = view;
this.waitTime = waitTime;
this.passedTime = 0;

// 創(chuàng)建并啟動(dòng)定時(shí)器

timer = new Timer(ONE_SECOND, new ActionListener()
{

public void actionPerformed(ActionEvent evt)
{
timeListener();
}
});
timer.start();

// 創(chuàng)建并啟動(dòng)線程來(lái)完成任務(wù)
thread = new Thread(this);
thread.start();
}


private void timeListener()
{
passedTime++;

// 動(dòng)態(tài)顯示狀態(tài)
int modSeed = passedTime % 3;

if (modSeed == 0)
{
view.getLabel2().setText("響應(yīng)中");

} else if (modSeed == 1)
{
view.getLabel2().setText("響應(yīng)中..");

} else if (modSeed == 2)
{
view.getLabel2().setText("響應(yīng)中
.");
}

// 如果流逝時(shí)間大于規(guī)定時(shí)間則中斷線程

if (passedTime > waitTime)
{
passedTime = waitTime;
thread.interrupt();
}
}


public void run()
{

while (passedTime < waitTime)
{

try
{
Thread.sleep(10000);// 模擬一個(gè)耗時(shí)相應(yīng)過(guò)程
timer.stop();// 任務(wù)完成,停止Timer

view.getLabel2().setText(model.getText2());

} catch (InterruptedException ex)
{
timer.stop();// 線程中斷,停止Timer
view.getLabel2().setText("在指定時(shí)間內(nèi)未響應(yīng)");

} catch (Exception ex)
{
ex.printStackTrace();
}

return;
}
}
}
執(zhí)行效果如下:
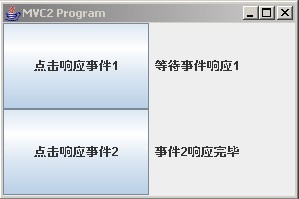
本文代碼下載(點(diǎn)擊第二個(gè)按鈕):
http://m.tkk7.com/Files/sitinspring/TimedThreadCallBack20071106194506.rar