? In the next several trails, we develop a new investment calculator application to demonstrate how to use EJB 3.0 entity beans. The new investment calculator allows you to save a list of fund company profiles and a list of individual investor profiles in a relational database. For each investment calculation, you can choose a company and an individual from the database, and then enter a monthly saving amount to calculate the final investment return. Each calculation record is also saved in the database with a time stamp. We can later search and update the calculation records in the database.
在下面的幾節中,我們將開發出一個全新的投資計算器以展示如何使用實體BEAN,這個新的投資計算器允許你在關系數據庫中保存一個fund company profiles列表和一個individual investor profiles列表。對于每一次的投資計算,你都可以從DB中選擇一個company和一個individual,然后輸入每月的存款來計算最終的投資回報。每一次的計算記錄用一個time stamp保存到DB中。這樣,我們可以在以后search和update這些記錄。
We construct the following object class hierarchy to model the data in the above investment calculator application. All classes conform to simple JavaBeans convention (i.e., standard getter and setter methods for data attributes).
我們將構建如下的object class體系來模擬上面所說的投資計算器。它們都遵循簡單的javabean規范(setter, getter).
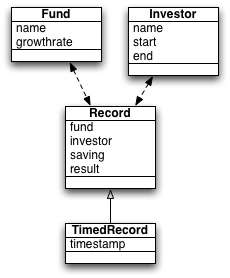
Fund類表示profiles of available investment funds。它有一個name和一個average annual growth rate屬性
Investor類表示profiles of individual investors.它有如下的數據屬性:投資者的name, 投資的start age, 投資的end age
Record類表示一次investment calculation。它有如下數據屬性:一個Fund,一個Investor,一個monthly saving amount, 還有一個final result.
TimedRecord類表示一次以time stamp標記的calculation record, 它繼承自Record類,并增加了一個timestamp類型的屬性。
@Entity
@Table(name?
=
?
"
fund
"
)
public
?
class
?Fund?
implements
?Serializable?{
??
private
?
int
?id;
??
private
?String?name;
??
private
?
double
?growthrate;
??
public
?Fund?()?{?}
??
public
?Fund?(String?name,?
double
?growthrate)?{
????
this
.name?
=
?name;
????
this
.growthrate?
=
?growthrate;
??}
??@Id
??@GeneratedValue
??
public
?
int
?getId?()?{
????
return
?id;
??}
??
public
?
void
?setId?(
int
?id)?{
????
this
.id?
=
?id;
??}
??
public
?String?getName?()?{
????
return
?name;
??}
??
public
?
void
?setName?(String?name)?{
????
this
.name?
=
?name;
??}
?
//
?Other?getter?and?setter?methods?
}
@Id 表示Id是表的主鍵
@GeneratedValue表示主鍵的值由Server自動生成。
你可以顯示的給每一個屬性指定它所對應的column,column的默認值同屬性的名字。
生成的schema如下圖所示:
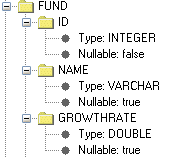
Investor類與Fund類似,這里不再表述。
Record實體bean比較Fund和Investor都要復雜,它不僅包括簡單的數據屬性,還包括其它bean(比如Fund和Investor)自身,為了表達它們之間的關系,在Record表中含有對應的外鍵(關聯到Fund和Investor的主鍵)。你可以在Record Class中使用標注@JoinColumn來表示這些外鍵,如果你忘了寫,EJB 3.0 server會給你指定一個默認的column.
標注還可以用來表示外鍵之間的約束關系。比如
@ManyToOne
@OneToMany
@OneToOne:
@ManyToMany
@Entity
@Table(name?
=
?
"
record
"
)
//
?
?
public
?
class
?Record?
implements
?Serializable?{
??
protected
?
int
?id;
??
protected
?Fund?fund;
??
protected
?Investor?investor;
??
protected
?
double
?saving;
??
protected
?
double
?result;
??
//
?
?
??@ManyToOne(optional
=
false
)
??@JoinColumn(name
=
"
my_fundid
"
)
??
public
?Fund?getFund?()?{
????
return
?fund;
??}
??@ManyToOne(optional
=
false
)
??
//
?Use?the?system-specified?join?column
??
public
?Investor?getInvestor?()?{
????
return
?investor;
??}
??
//
?Other?getter?and?setter?methods?
}
TimedRecord類繼承Record,有多種方式映射繼承關系。比如,你可以給繼承體系中的每一個類一個獨立的表然后使用外鍵來約束它們之間的關系。在這個示例中,我們使用另外一種映射策略---單表映射策略。
single table mapping strategy是EJB3.0中默認的繼承映射策略。它把基類Record和子類TimedRecord映射到同一張表。這張表中含有所有類中的所有屬性。用一個特殊的列來區分是哪一個子類。
必須在基類中指定Mapping Strategy和differentiator column !我們分別用@DiscriminatorColumn 和 @DiscriminatorValue來標注,在這個例子中,Record表中的 record_type表示 differentiator, 如果它的值是B,則表示它是基類Record
@Entity
@Table(name?
=
?
"
record
"
)
@DiscriminatorColumn(name
=
"
record_type
"
)
@DiscriminatorValue(value
=
"
B
"
)
public
?
class
?Record?{
????
//
?
?
}
TimedRecord 類簡單的繼承自 Record class.它的@DiscriminatorValue表明所有的TimedRecord在record_type這一列上的值為T
@Entity
@DiscriminatorValue?(value
=
"
T
"
)
public
?
class
?TimedRecord?
extends
?Record?{
??
private
?Timestamp?ts;
??
//
?
?
??
public
?Timestamp?getTs?()?{
????
return
?ts;
??}
??
public
?
void
?setTs?(Timestamp?ts)?{
????
this
.ts?
=
?ts;
??}
}