增加一些內容
現在我們已經有一個view能夠成功運行了.我們可以往里面增加一
public void createPartControl(Composite parent) {
toolkit = new FormToolkit(parent.getDisplay());
form = toolkit.createForm(parent);
form.setText("Hello, Eclipse Forms");
GridLayout layout = new GridLayout();
form.getBody().setLayout(layout);
Hyperlink link = toolkit.createHyperlink(form.getBody(),
"Click here.", SWT.WRAP);
link.addHyperlinkListener(new HyperlinkAdapter() {
public void linkActivated(HyperlinkEvent e) {
System.out.println("Link activated!");
}
});
}
form的body是標題下面的可用空間.因為這個空間是一個SW我們為body設置了layout,
然后創建了一個超鏈接.超鏈接是由Eclipse Forms提供的為數不多的組件之一.我們可以為超鏈接
增加監聽器,這樣能夠在用戶點擊它時做出反應.
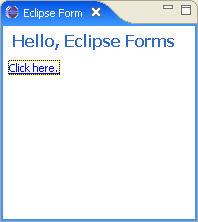
超鏈接組(Hyperlink Groups)
Form tookit有一個"超鏈接組"對象.每個創建出的超鏈接都加入這個組對象中.超鏈接為多個角色服
當你要改變超鏈接組對象的默認設置時,可以通過toolkit的g
創建普通組件
layout.numColumns = 2;
GridData gd = new GridData();
gd.horizontalSpan = 2;
link.setLayoutData(gd);
Label label = new Label(form.getBody(), SWT.NULL);
label.setText("Text field label:");
Text text = new Text(form.getBody(), SWT.BORDER);
text.setLayoutData(new GridData(GridData.FILL_HORIZONTAL));
Button button = new Button(form.getBody(), SWT.CHECK);
button.setText("An example of a checkbox in a form");
gd = new GridData();
gd.horizontalSpan = 2;
button.setLayoutData(gd);
現在我們使用了兩列,并且創建了一個標簽(label)
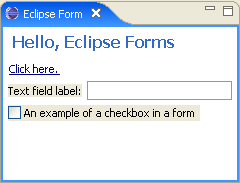
圖4:一個擁有直接用它們的構造器創建出的SWT組件的form
Label label = toolkit.createLabel(form.getBody(), "Text field label:");
Text text = toolkit.createText(form.getBody(), "");
text.setLayoutData(new GridData(GridData.FILL_HORIZONTAL));
Button button = toolkit.createButton(form.getBody(), "A checkbox in a form", SWT.CHECK);
gd = new GridData();
gd.horizontalSpan = 2;
button.setLayoutData(gd);
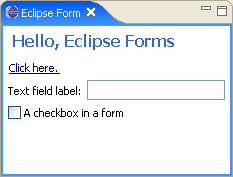
圖5:一個擁有用form toolkit的工廠方法創建出的SWT組件的form
由form toolkit提供的工廠方法是為了方便.Toolkit沒有函蓋
達到"平滑"的視覺效果
一個在PDE編輯器中Eclipse Forms的可看出的屬性是組件的"平滑"視覺效果.以前所有沒有3D邊框的組件在窗口中看起來不錯,但是在編輯器或視圖中就不行.這個支持寫在FormToolkit
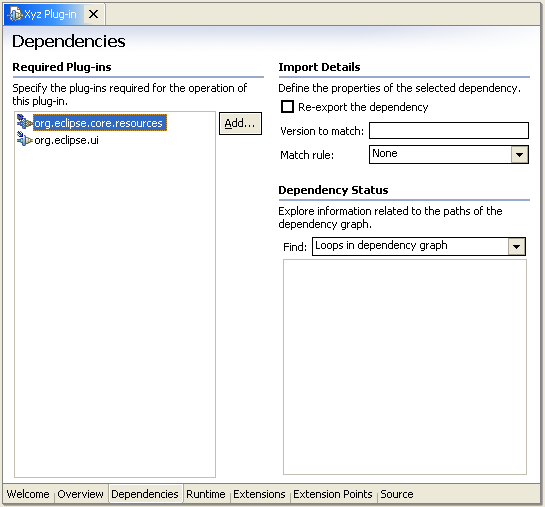
象表格,文本類,復選框等,是加上一個平滑的1個象素寬的邊框.這些邊框不是來自組件自己(SWT.BORDER風格沒有用到).另外,如果設置一下,tookit會為每個組件的parent
Form toolkit知道哪個組件需要一個定制的邊框.但是,你可能新創建了一個不在原來名單中的組件,它也需要一個邊框.你可以通過象下面這樣的代碼給toolkit一
Control myControl = new MyControl(parent);
myControl.setData(FormToolkit.KEY_DRAW_BORDER, FormToolkit.TEXT_BORDER);
// or myControl.setData(FormToolkit.KEY_DRAW_BORDER, FormToolkit.TREE_BORDER);
toolkit.paintBordersFor(parent);
你可以在上面這張圖中看出,象樹和表格這樣的"結構化
因為Eclipse 3.0和在Windows XP上,當javaw.exe.manifest文件在Java虛擬機bi
定制布局(Custom layouts)
Eclipse Forms在SWT layout的基礎上增加了兩個新的layout
現在我們知道如何來組合一個form,讓我們先給一個懸念.我們會改變那個超鏈接文本使它加長一些:
link.setText("This is an example of a form that is much longer "+
"and will need to wrap.");
讓我們看看結果:
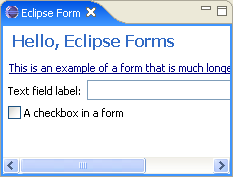
圖片7:一個使用GridLayout的form
發生什么事了?記住我們使用的是GridLayout
我們需要的是一個象HTML表格一樣的layout
但是,它們在布局時完全不同.TableWrapLayout從列開始
public void createPartControl(Composite parent) {
toolkit = new FormToolkit(parent.getDisplay());
form = toolkit.createForm(parent);
form.setText("Hello, Eclipse Forms");
TableWrapLayout layout = new TableWrapLayout();
form.getBody().setLayout(layout);
Hyperlink link = toolkit.createHyperlink(form.getBody(),"Click here.", SWT.WRAP);
link.addHyperlinkListener(new HyperlinkAdapter() {
public void linkActivated(HyperlinkEvent e) {
System.out.println("Link activated!");
}
});
link.setText("This is an example of a form that is much longer and will need to wrap.");
layout.numColumns = 2;
TableWrapData td = new TableWrapData();
td.colspan = 2;
link.setLayoutData(td);
Label label = toolkit.createLabel(form.getBody(), "Text field label:");
Text text = toolkit.createText(form.getBody(), "");
td = new TableWrapData(TableWrapData.FILL_GRAB);
text.setLayoutData(td);
Button button = toolkit.createButton(form.getBody(), "A checkbox in a form", SWT.CHECK);
td = new TableWrapData();
td.colspan = 2;
button.setLayoutData(td);
}
我們用了GridData相同的概念.一些變量擁有不同的名字(舉個例子,colspan和rowspan,align和valign取自HTML TABLE的屬性),但是你可以做相同的事--創建一個超鏈接和按鈕占據兩列的格子 .因為空白(margins)和GridLayout是相同的 ,結果會看起來一樣,除了超鏈接現在會包裹起來:
圖片8:一個使用TableWrapLayout的form
TableWrapLayout和GridLayout的一個主要
你也許會注意到一個地方和剛剛說的不符:TableWrapLay
為了用TableWrapLayout有好的結果
public interface ILayoutExtension {
/**
* Computes the minimum width of the parent. All widgets capable of word
* wrapping should return the width of the longest word that cannot be
* broken any further.
*
* @param parent the parent composite
* @param changed <code>true</code> if the cached information should be
* flushed, <code>false</code> otherwise.
* @return the minimum width of the parent composite
*/
public int computeMinimumWidth(Composite parent, boolean changed);
/**
* Computes the maximum width of the parent. All widgets capable of word
* wrapping should return the length of the entire text with wrapping
* turned off.
*
* @param parent the parent composite
* @param changed <code>true</code> if the cached information
* should be flushed, <code>false</code> otherwise.
* @return the maximum width of the parent composite
*/
public int computeMaximumWidth(Composite parent, boolean changed);
}
TableWrapLayout本身實現了這個接口,這樣讓它處理當composites的layout是這個comp
layout.numColumns = 3;
Label label;
TableWrapData td;
label = toolkit.createLabel(form.getBody(),
"Some text to put in the first column", SWT.WRAP);
label = toolkit.createLabel(form.getBody(),
"Some text to put in the second column and make it a bit "+
"longer so that we can see what happens with column "+
distribution. This text must be the longest so that it can "+
"get more space allocated to the columns it belongs to.",
SWT.WRAP);
td = new TableWrapData();
td.colspan = 2;
label.setLayoutData(td);
label = toolkit.createLabel(form.getBody(),
"This text will span two rows and should not grow the column.",
SWT.WRAP);
td = new TableWrapData();
td.rowspan = 2;
label.setLayoutData(td);
label = toolkit.createLabel(form.getBody(),
"This text goes into column 2 and consumes only one cell",
SWT.WRAP);
label.setLayoutData(new TableWrapData(TableWrapData.FILL_GRAB));
label = toolkit.createLabel(form.getBody(),
"This text goes into column 3 and consumes only one cell too",
SWT.WRAP);
label.setLayoutData(new TableWrapData(TableWrapData.FILL));
label = toolkit.createLabel(form.getBody(),
"This text goes into column 2 and consumes only one cell",
SWT.WRAP);
label.setLayoutData(new TableWrapData(TableWrapData.FILL_GRAB));
label = toolkit.createLabel(form.getBody(),
"This text goes into column 3 and consumes only one cell too",
SWT.WRAP);
label.setLayoutData(new TableWrapData(TableWrapData.FILL));form.getBody().setBackground(form.getBody().getDisplay().
getSystemColor(SWT.COLOR_WIDGET_BACKGROUND));
這個創建了一些有不同長度文本的label.有些labels占據多列,有些占據多行.為了讓測試更加簡單,
我們把form的背景設置為組件背景,這樣單元能夠更簡單看出來.當我們運行例子,我們會得到下面的樣子:
圖片9:TableWrapLayout留下的多余空間
關鍵之處在于組件最小寬度和最大寬度相比較時.相差越多,結果越明顯,會看到列中有很大的縫隙.占據的寬度是組件所需要的最小的寬度
ColumnLayout
Eclipse Forms另外一個定制的layout是SWT RowLayout的變種.如果我們把RowLayout上的ch
在更加復雜的forms中我們需要列的數量按情況變化.換句話來說,我們希望數字按照form的寬度來改變--有可能需要更多的列,當寬度減小時則把數字減小.而且我們希望所有列的長度象報紙布局一
與TableWrapLayout相比,ColumnLayout更加簡單.不需要復雜的設置
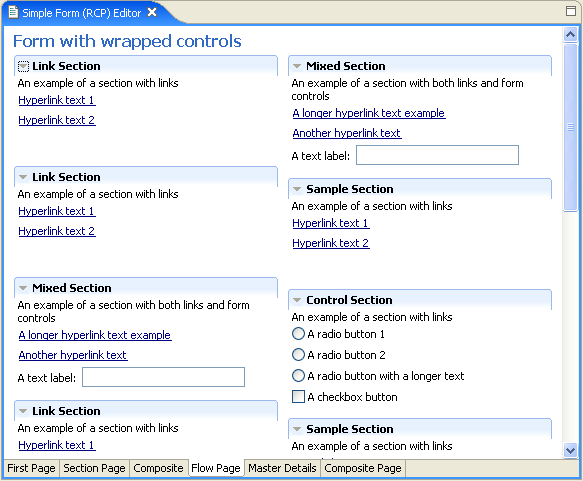
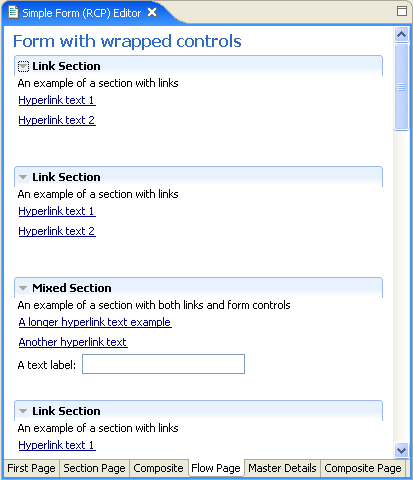
轉自:http://blog.csdn.net/starshus/archive/2006/02/07/593785.aspx